Stack and Queues
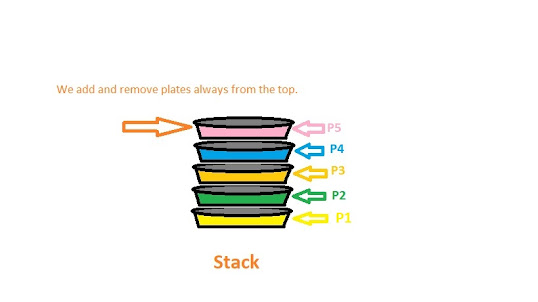
What is Stack? The basis of stack is first in last out(FILO) Or last in first out (LIFO). To understand stack, consider example of plats. We place plats one over another. Let say we have 5 plats, named as p1, p2, p3, p4, and p5. Assume they are arranged in such a way that p2 is placed on p1, p3 is placed on p2, p4 on p3, and p5 on p4. As shown in the diagram. Now you are clear with, what is stack. Now we will see where it is used and how we implement stack in c plus plus programming language. Why Stack? We see the functionality of forward and backward operations in our browsers. Similarly, we see functionalities like undo, redo in our text editor or any application like photo editors. The functionality of undo, redo is very common. It is used in many applications like in word, excel, photoshop, browsers, paint, and many other applications. Now if we think about what will be the best way to implement undo, redo functionality in our application, we will see the best data structure ...